深入了解AppLauncher#
在本教程中,我们将深入研究 app.AppLauncher
类,以使用CLI参数和环境变量(envars)配置模拟器。特别地,我们将展示如何使用 AppLauncher
启用实时流传输,并配置其包装的 omni.isaac.kit.SimulationApp
实例,同时也允许用户提供选项。
AppLauncher
是 SimulationApp
的一个包装器,用于简化其配置。 SimulationApp
有许多扩展功能,必须加载才能启用不同的功能,并且其中一些扩展功能是有顺序和相互依赖的。此外,还有启动选项,比如 headless
必须在实例化时设置,并且这些选项与某些扩展功能有暗含的关联,比如实时流传输扩展功能。 AppLauncher
提供了一个接口,可以以便捷的方式处理这些扩展功能和启动选项,适用于各种用例。为此,我们提供了CLI和envar标志,可以与用户定义的CLI参数合并,同时传递给 SimulationApp
。
代码#
该教程对应于 source/standalone/tutorials/00_sim
目录中的 launch_app.py
脚本。
launch_app.py的代码
1# Copyright (c) 2022-2025, The Isaac Lab Project Developers.
2# All rights reserved.
3#
4# SPDX-License-Identifier: BSD-3-Clause
5
6"""
7This script demonstrates how to run IsaacSim via the AppLauncher
8
9.. code-block:: bash
10
11 # Usage
12 ./isaaclab.sh -p source/standalone/tutorials/00_sim/launch_app.py
13
14"""
15
16"""Launch Isaac Sim Simulator first."""
17
18
19import argparse
20
21from omni.isaac.lab.app import AppLauncher
22
23# create argparser
24parser = argparse.ArgumentParser(description="Tutorial on running IsaacSim via the AppLauncher.")
25parser.add_argument("--size", type=float, default=1.0, help="Side-length of cuboid")
26# SimulationApp arguments https://docs.omniverse.nvidia.com/py/isaacsim/source/extensions/omni.isaac.kit/docs/index.html?highlight=simulationapp#omni.isaac.kit.SimulationApp
27parser.add_argument(
28 "--width", type=int, default=1280, help="Width of the viewport and generated images. Defaults to 1280"
29)
30parser.add_argument(
31 "--height", type=int, default=720, help="Height of the viewport and generated images. Defaults to 720"
32)
33
34# append AppLauncher cli args
35AppLauncher.add_app_launcher_args(parser)
36# parse the arguments
37args_cli = parser.parse_args()
38# launch omniverse app
39app_launcher = AppLauncher(args_cli)
40simulation_app = app_launcher.app
41
42"""Rest everything follows."""
43
44import omni.isaac.lab.sim as sim_utils
45
46
47def design_scene():
48 """Designs the scene by spawning ground plane, light, objects and meshes from usd files."""
49 # Ground-plane
50 cfg_ground = sim_utils.GroundPlaneCfg()
51 cfg_ground.func("/World/defaultGroundPlane", cfg_ground)
52
53 # spawn distant light
54 cfg_light_distant = sim_utils.DistantLightCfg(
55 intensity=3000.0,
56 color=(0.75, 0.75, 0.75),
57 )
58 cfg_light_distant.func("/World/lightDistant", cfg_light_distant, translation=(1, 0, 10))
59
60 # spawn a cuboid
61 cfg_cuboid = sim_utils.CuboidCfg(
62 size=[args_cli.size] * 3,
63 visual_material=sim_utils.PreviewSurfaceCfg(diffuse_color=(1.0, 1.0, 1.0)),
64 )
65 # Spawn cuboid, altering translation on the z-axis to scale to its size
66 cfg_cuboid.func("/World/Object", cfg_cuboid, translation=(0.0, 0.0, args_cli.size / 2))
67
68
69def main():
70 """Main function."""
71
72 # Initialize the simulation context
73 sim_cfg = sim_utils.SimulationCfg(dt=0.01, device=args_cli.device)
74 sim = sim_utils.SimulationContext(sim_cfg)
75 # Set main camera
76 sim.set_camera_view([2.0, 0.0, 2.5], [-0.5, 0.0, 0.5])
77
78 # Design scene by adding assets to it
79 design_scene()
80
81 # Play the simulator
82 sim.reset()
83 # Now we are ready!
84 print("[INFO]: Setup complete...")
85
86 # Simulate physics
87 while simulation_app.is_running():
88 # perform step
89 sim.step()
90
91
92if __name__ == "__main__":
93 # run the main function
94 main()
95 # close sim app
96 simulation_app.close()
代码解释#
向argparser添加参数#
AppLauncher
旨在与用户自定义脚本需要的自定义CLI参数兼容,同时提供便携CLI界面。
在本教程中,实例化了一个标准的 argparse.ArgumentParser
,并赋予了特定脚本的 --size
参数,以及参数 --height
和 --width
。后者被 SimulationApp
所列取。
参数 --size
不被 AppLauncher
使用,但会与 AppLauncher
界面无缝合并。可以通过 add_app_launcher_args()
方法将脚本参数与 AppLauncher
界面合并,该方法会返回一个修改过的 ArgumentParser
,其中包含 AppLauncher
参数。然后可以使用标准的 argparse.ArgumentParser.parse_args()
方法将其处理为一个 argparse.Namespace
,并直接传递给 AppLauncher
进行实例化。
import argparse
from omni.isaac.lab.app import AppLauncher
# create argparser
parser = argparse.ArgumentParser(description="Tutorial on running IsaacSim via the AppLauncher.")
parser.add_argument("--size", type=float, default=1.0, help="Side-length of cuboid")
# SimulationApp arguments https://docs.omniverse.nvidia.com/py/isaacsim/source/extensions/omni.isaac.kit/docs/index.html?highlight=simulationapp#omni.isaac.kit.SimulationApp
parser.add_argument(
"--width", type=int, default=1280, help="Width of the viewport and generated images. Defaults to 1280"
)
parser.add_argument(
"--height", type=int, default=720, help="Height of the viewport and generated images. Defaults to 720"
)
# append AppLauncher cli args
AppLauncher.add_app_launcher_args(parser)
# parse the arguments
args_cli = parser.parse_args()
# launch omniverse app
app_launcher = AppLauncher(args_cli)
simulation_app = app_launcher.app
上述仅演示了一种向 AppLauncher
传递参数的方式。请查阅其文档页面以获取更多选项。
理解–help的输出#
在执行脚本时,我们可以传递 --help
参数,并查看自定义参数和 AppLauncher
的合并输出。
./isaaclab.sh -p source/standalone/tutorials/00_sim/launch_app.py --help
[INFO] Using python from: /isaac-sim/python.sh
[INFO][AppLauncher]: The argument 'width' will be used to configure the SimulationApp.
[INFO][AppLauncher]: The argument 'height' will be used to configure the SimulationApp.
usage: launch_app.py [-h] [--size SIZE] [--width WIDTH] [--height HEIGHT] [--headless] [--livestream {0,1,2}]
[--enable_cameras] [--verbose] [--experience EXPERIENCE]
Tutorial on running IsaacSim via the AppLauncher.
options:
-h, --help show this help message and exit
--size SIZE Side-length of cuboid
--width WIDTH Width of the viewport and generated images. Defaults to 1280
--height HEIGHT Height of the viewport and generated images. Defaults to 720
app_launcher arguments:
--headless Force display off at all times.
--livestream {0,1,2}
Force enable livestreaming. Mapping corresponds to that for the "LIVESTREAM" environment variable.
--enable_cameras Enable cameras when running without a GUI.
--verbose Enable verbose terminal logging from the SimulationApp.
--experience EXPERIENCE
The experience file to load when launching the SimulationApp.
* If an empty string is provided, the experience file is determined based on the headless flag.
* If a relative path is provided, it is resolved relative to the `apps` folder in Isaac Sim and
Isaac Lab (in that order).
该输出详细描述了脚本中直接定义的 --size
、--height
和 --width
参数,以及 AppLauncher
的参数。
在帮助输出之前的 [INFO]
消息还指出了这些参数将被解释为 AppLauncher
类包装的 SimulationApp
实例的参数。在这种情况下,它是 --height
和 --width
。这两个参数被归类为这样的类别,因为它们与 SimulationApp
可以处理的参数的名称和类型相匹配。请参考 specification 以获取更多示例。
使用环境变量#
如帮助信息中所述, AppLauncher
的参数( --livestream
、--headless
)有相应的环境变量(envar)。这些在 omni.isaac.lab.app
文档中详细说明。通过CLI提供这些参数等同于在设置了相应envar的shell环境中运行脚本。
支持 AppLauncher
envar只是为了提供会话持久性配置的便利,并可以在用户的 ${HOME}/.bashrc
中设置,以在会话之间保留设置。在这些参数从CLI提供的情况下,它们将覆盖其相应的envar,后面我们将在本教程中演示。
这些参数可以与使用 AppLauncher
启动模拟的任何脚本一起使用,只有一个例外,即 --enable_cameras
。此设置将设置渲染管线以使用离屏渲染器。但是,这个设置仅与 omni.isaac.lab.sim.SimulationContext
兼容。它将无法与Isaac Sim的 omni.isaac.core.simulation_context.SimulationContext
类一起使用。有关此标记的更多信息,请参阅 AppLauncher
的API文档。
代码执行#
现在我们将运行示例脚本:
LIVESTREAM=1 ./isaaclab.sh -p source/standalone/tutorials/00_sim/launch_app.py --size 0.5
这将在模拟中生成一个0.5立方米的体积长方体。不会出现GUI,等同于如果我们传递了 --headless
标志,因为无头模式是由我们的 LIVESTREAM
envar隐含的。如果需要可视化,可以通过Isaac的 Native Livestreaming 来实现。目前,流传输是容器内可视化的唯一支持方法。可以通过在启动终端中按 Ctrl+C
键终止该进程。
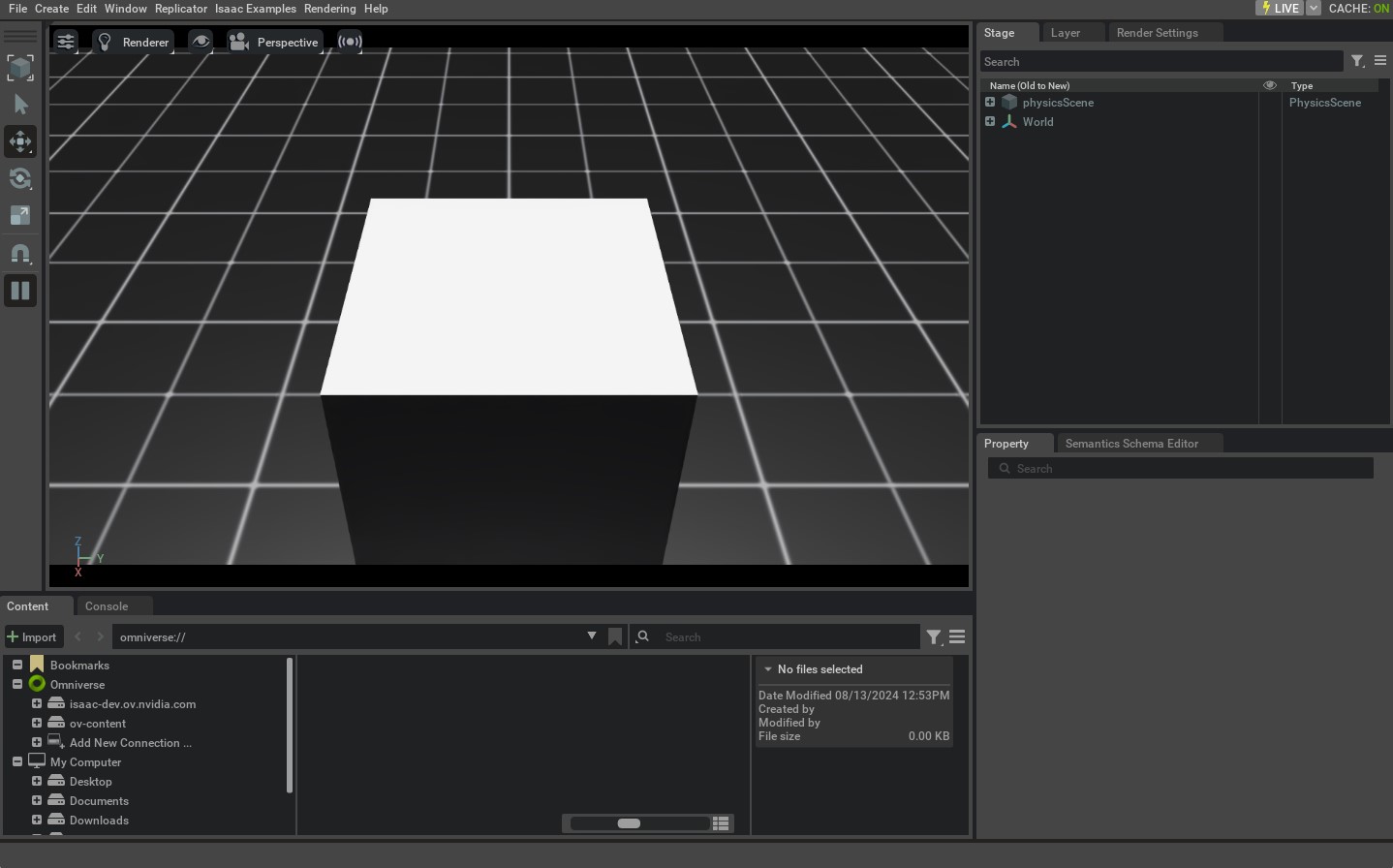
现在,让我们看看 AppLauncher
如何处理冲突命令:
LIVESTREAM=0 ./isaaclab.sh -p source/standalone/tutorials/00_sim/launch_app.py --size 0.5 --livestream 1
这会导致与上次运行相同的行为,因为虽然我们在envars中设置了 LIVESTREAM=0
,但CLI参数如 --livestream
优先确定行为。可以通过在启动终端中按 Ctrl+C
键来终止该进程。
最后,我们将研究通过 AppLauncher
向 SimulationApp
传递参数:
LIVESTREAM=1 ./isaaclab.sh -p source/standalone/tutorials/00_sim/launch_app.py --size 0.5 --width 1920 --height 1080
这将导致与之前的行为相同,但现在视口将以1920x1080p分辨率进行渲染。在希望收集高分辨率视频或者希望使模拟更具性能时,这可能非常有用。可以通过在启动终端中按 Ctrl+C
键来终止该进程。