创建一个空场景#
本教程展示了如何从独立的 Python 脚本启动和控制 Isaac Sim 模拟器。它在 Isaac Lab 中设置了一个空场景,并介绍了框架中使用的两个主要类 app.AppLauncher
和 sim.SimulationContext
。
请在开始本教程之前查看 Isaac Sim 工作流 ,以获得对与模拟器一起工作的初步了解。
代码#
本教程对应于 scripts/tutorials/00_sim
目录中的 create_empty.py
脚本。
create_empty.py的代码
1# Copyright (c) 2022-2025, The Isaac Lab Project Developers (https://github.com/isaac-sim/IsaacLab/blob/main/CONTRIBUTORS.md).
2# All rights reserved.
3#
4# SPDX-License-Identifier: BSD-3-Clause
5
6"""This script demonstrates how to create a simple stage in Isaac Sim.
7
8.. code-block:: bash
9
10 # Usage
11 ./isaaclab.sh -p scripts/tutorials/00_sim/create_empty.py
12
13"""
14
15"""Launch Isaac Sim Simulator first."""
16
17
18import argparse
19
20from isaaclab.app import AppLauncher
21
22# create argparser
23parser = argparse.ArgumentParser(description="Tutorial on creating an empty stage.")
24# append AppLauncher cli args
25AppLauncher.add_app_launcher_args(parser)
26# parse the arguments
27args_cli = parser.parse_args()
28# launch omniverse app
29app_launcher = AppLauncher(args_cli)
30simulation_app = app_launcher.app
31
32"""Rest everything follows."""
33
34from isaaclab.sim import SimulationCfg, SimulationContext
35
36
37def main():
38 """Main function."""
39
40 # Initialize the simulation context
41 sim_cfg = SimulationCfg(dt=0.01)
42 sim = SimulationContext(sim_cfg)
43 # Set main camera
44 sim.set_camera_view([2.5, 2.5, 2.5], [0.0, 0.0, 0.0])
45
46 # Play the simulator
47 sim.reset()
48 # Now we are ready!
49 print("[INFO]: Setup complete...")
50
51 # Simulate physics
52 while simulation_app.is_running():
53 # perform step
54 sim.step()
55
56
57if __name__ == "__main__":
58 # run the main function
59 main()
60 # close sim app
61 simulation_app.close()
代码解释#
启动模拟器#
使用独立的 Python 脚本时的第一步是启动模拟应用程序。这是必要的,因为只有在模拟应用程序运行后,Isaac Sim 的各种依赖模块才可用。
这可以通过导入 app.AppLauncher
类来完成。此实用程序类包装了 isaacsim.SimulationApp
类,以启动模拟器。它提供机制来使用命令行参数和环境变量配置模拟器。
对于本教程,我们主要关注将命令行选项添加到用户定义的 argparse.ArgumentParser
中。通过将解析器实例传递给 app.AppLauncher.add_app_launcher_args()
方法,并向其附加不同的参数来完成。这些参数包括启动应用程序无头模式、配置不同的实时流选项和启用脱机渲染。
import argparse
from isaaclab.app import AppLauncher
# create argparser
parser = argparse.ArgumentParser(description="Tutorial on creating an empty stage.")
# append AppLauncher cli args
AppLauncher.add_app_launcher_args(parser)
# parse the arguments
args_cli = parser.parse_args()
# launch omniverse app
app_launcher = AppLauncher(args_cli)
simulation_app = app_launcher.app
导入 python 模块#
一旦模拟应用程序运行,就可以从 Isaac Sim 和其他库中导入不同的 Python 模块。在这里,我们导入以下模块:
isaaclab.sim
:Isaac Lab 中用于所有核心模拟器相关操作的子包。
from isaaclab.sim import SimulationCfg, SimulationContext
配置模拟上下文#
当从独立脚本启动模拟器时,用户可以完全控制播放、暂停和步进模拟器。所有这些操作都通过 模拟上下文 处理。它负责各种时间轴事件,并为模拟器配置 物理场景 。
在 Isaac Lab 中 , sim.SimulationContext
类继承了 Isaac Sim 的 isaacsim.core.api.simulation_context.SimulationContext
,以允许通过 Python 的 dataclass
对象配置模拟器,并处理模拟步进的某些复杂性。
对于本教程,我们将将物理和渲染时间步长设置为0.01秒。通过将这些数量传递给 sim.SimulationCfg
,然后用它创建模拟上下文的实例。
# Initialize the simulation context
sim_cfg = SimulationCfg(dt=0.01)
sim = SimulationContext(sim_cfg)
# Set main camera
sim.set_camera_view([2.5, 2.5, 2.5], [0.0, 0.0, 0.0])
创建模拟上下文后,我们只配置了作用于模拟场景的物理。这包括用于模拟的设备、重力矢量和其他高级求解器参数。现在还有两个主要步骤剩下来运行模拟:
设计模拟场景: 添加传感器、机器人和其他模拟对象
运行模拟循环: 使模拟器进行步进,并从模拟器中设置和获取数据
在本教程中,我们首先看Step 2的空场景,以便首先关注模拟控制。在以下教程中,我们将深入研究Step 1和使用模拟处理程序与模拟器进行交互。
运行模拟#
在设置模拟场景之后,第一件事是调用 sim.SimulationContext.reset()
方法。此方法播放时间轴并初始化模拟器中的物理处理。在第一次步进模拟器之前必须始终调用此方法,否则模拟处理不会正确初始化。
备注
sim.SimulationContext.reset()
不同于 sim.SimulationContext.play()
方法,因为后者只播放时间轴而不初始化物理处理。
播放模拟时间轴后,我们设置了一个简单的模拟循环,其中模拟器在模拟应用程序运行时重复进行步进。 sim.SimulationContext.step()
方法带有 render
作为参数,决定步进是否包括更新与渲染相关的事件。默认情况下,此标志设置为True。
# Play the simulator
sim.reset()
# Now we are ready!
print("[INFO]: Setup complete...")
# Simulate physics
while simulation_app.is_running():
# perform step
sim.step()
退出模拟#
最后,通过调用 isaacsim.SimulationApp.close()
方法停止模拟应用程序并关闭其窗口。
# close sim app
simulation_app.close()
代码执行#
现在我们已经浏览了代码,让我们运行脚本并查看结果:
./isaaclab.sh -p scripts/tutorials/00_sim/create_empty.py
模拟应该正在进行,并且场景应该正在渲染。要停止模拟,可以关闭窗口或在终端中按下 Ctrl+C
。
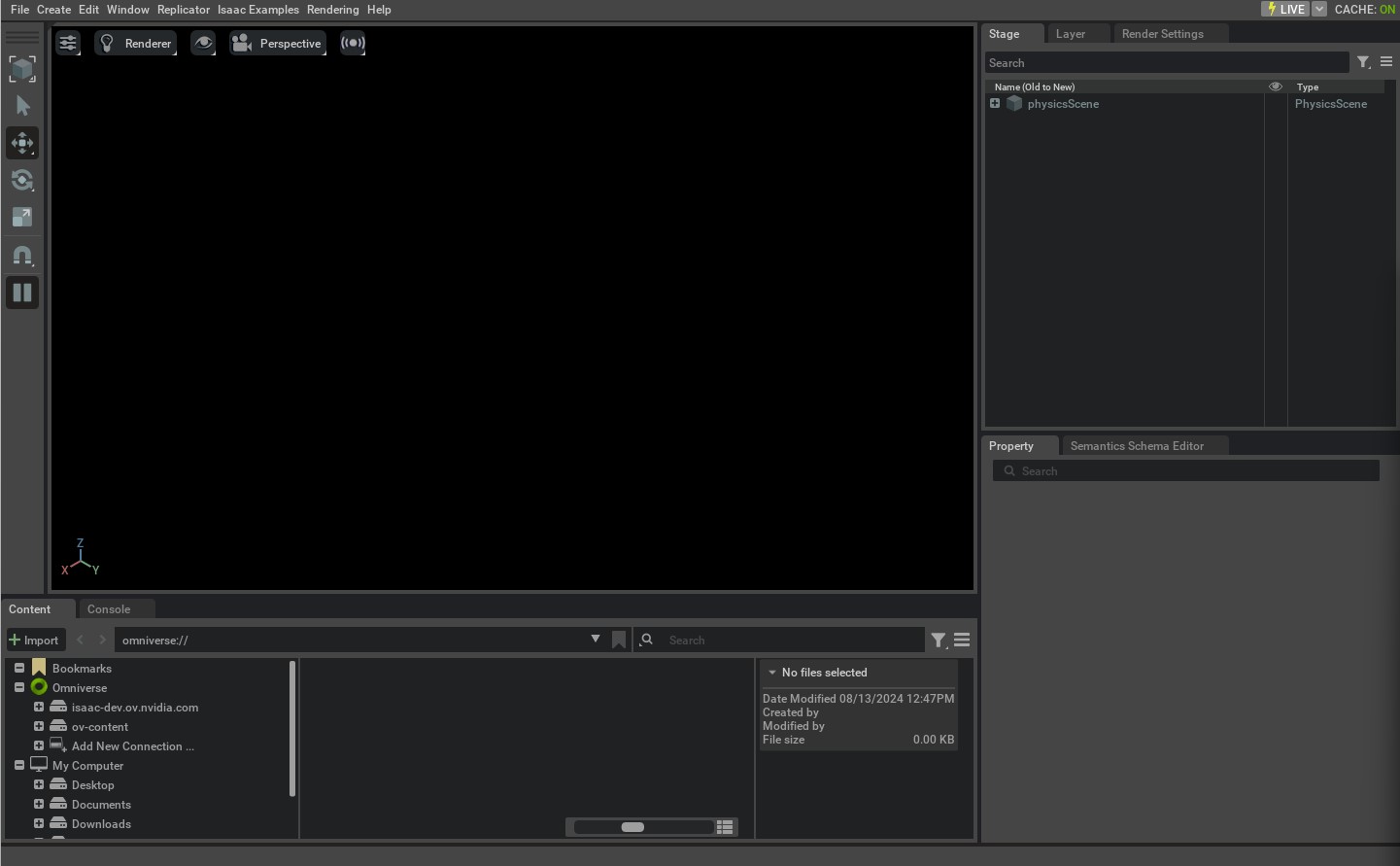
将 --help
传递给上述脚本将显示先前由 app.AppLauncher
类添加的不同命令行参数。要以无头模式运行脚本,可以执行以下操作:
./isaaclab.sh -p scripts/tutorials/00_sim/create_empty.py --headless
现在我们对如何运行模拟有了基本的了解,让我们转到下一个教程,在那里我们将学习如何将资产添加到场景。